Matrix/TensorFlow for Baby Hawk: Difference between revisions
Jump to navigation
Jump to search
(Created page with "thumb|left|alt=Papa and Baby Hawk == Integrating Baby Hawk (Gemini 1.5) with Matrix Group Chat and TensorFlow in Docker == This tutorial will guide you through setting up a Gemini 1.5 API to interact in various Matrix rooms, while leveraging TensorFlow model running in a Docker container with Jupyter Notebook. === Prerequisites === * Running instance of Baby Hawk's Gemini 1.5 API on `ailounge.xyz` * TensorFlow instance running in Docker with J...") |
|||
(27 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
[[File:Papa-n-baby.jpg| | [[File:Papa-n-baby.jpg|600px|right|alt=Papa and Baby Hawk]] | ||
= Integrating Baby Hawk (Gemini 1.5) with Matrix Group Chat, TensorFlow in Docker, and PostgreSQL = | |||
This tutorial | This tutorial guides you through integrating a Gemini 1.5 API to interact in various Matrix rooms, leveraging a TensorFlow model running in a Docker container equipped with Jupyter Notebook, and securing communications using SSL, with the addition of a PostgreSQL database. | ||
== Prerequisites == | |||
* Running instance of Baby Hawk's Gemini 1.5 API on `ailounge.xyz` | * Running instance of Baby Hawk's Gemini 1.5 API on `ailounge.xyz` | ||
* TensorFlow instance running in Docker with Jupyter Notebook | * TensorFlow instance running in Docker with Jupyter Notebook | ||
* Matrix Synapse server running in Docker | * Matrix Synapse server running in Docker | ||
* Basic knowledge of Docker, Python, Jupyter Notebooks, and Matrix | * PostgreSQL database running in Docker | ||
* Basic knowledge of Docker, Python, Jupyter Notebooks, PostgreSQL, and Matrix | |||
* Domain names set up for `matrix.ailounge.xyz` and Jupyter accessed via `ailounge.xyz` | |||
== Step 1: Set Up Matrix Synapse Server using Docker == | |||
# '''Install Docker and Docker Compose:''' If not already installed, install Docker and Docker Compose. | |||
# '''Create a Docker Compose File (docker-compose.yml):''' | |||
<source lang="yaml"> | |||
version: '3' | version: '3' | ||
services: | services: | ||
postgres: | |||
image: postgres:latest | |||
restart: always | |||
environment: | |||
POSTGRES_DB: matrix_synapse | |||
POSTGRES_USER: matrix_user | |||
POSTGRES_PASSWORD: your_secure_password | |||
volumes: | |||
- ./postgres-data:/var/lib/postgresql/data | |||
ports: | |||
- "5432:5432" | |||
synapse: | synapse: | ||
image: matrixdotorg/synapse:latest | image: matrixdotorg/synapse:latest | ||
Line 24: | Line 35: | ||
environment: | environment: | ||
- SYNAPSE_SERVER_NAME=matrix.ailounge.xyz | - SYNAPSE_SERVER_NAME=matrix.ailounge.xyz | ||
- SYNAPSE_REPORT_STATS=yes | - SYNAPSE_REPORT_STATS=yes | ||
- SYNAPSE_DATABASE_CONFIG=postgres://matrix_user:your_secure_password@postgres/matrix_synapse | |||
depends_on: | |||
- postgres | |||
volumes: | volumes: | ||
- ./data:/data | - ./data:/data | ||
ports: | ports: | ||
- 8008:8008 | - 8008:8008 | ||
- 8448:8448 | - 8448:8448 | ||
</source> | |||
# '''Run Docker Compose:''' | |||
<source lang="bash"> | |||
docker-compose up -d | docker-compose up -d | ||
</source> | |||
# '''Register Admin User:''' Follow instructions in the container logs to register an admin user. | |||
== Step 2: Secure Jupyter Notebook and Matrix Synapse with SSL Using Certbot == | |||
# '''Install Certbot:''' | |||
<source lang="bash"> | |||
sudo apt install certbot | |||
</source> | |||
# '''Obtain Certificates:''' | |||
<source lang="bash"> | |||
sudo certbot certonly --standalone -d ailounge.xyz -d matrix.ailounge.xyz | |||
</source> | |||
# '''Configure Jupyter to Use SSL:''' | |||
Add SSL configuration to the Docker command for Jupyter: | |||
<source lang="bash"> | |||
--NotebookApp.certfile=/etc/ssl/certs/jupyter.crt --NotebookApp.keyfile=/etc/ssl/private/jupyter.key | |||
</source> | |||
# '''Configure Matrix Synapse to Use SSL:''' | |||
Update Synapse's configuration to use the obtained SSL certificates. | |||
== Step 3: Configure and Run Baby Hawk's Gemini 1.5 API == | |||
Ensure the API endpoint for Baby Hawk is publicly accessible and secured with SSL. | |||
3 | |||
5 | |||
== Step 4: Implement Continuous Learning with TensorFlow in Docker == | |||
# '''Expose Ports:''' Ensure the TensorFlow container exposes port 8888. | |||
# '''Install Libraries:''' Within the container, install libraries needed for integration, e.g., `matrix-nio`. | |||
# '''Access MongoDB:''' Configure access to MongoDB for data storage. | |||
# '''Collect & Preprocess Data:''' Use Jupyter Notebook for data handling. | |||
# '''Retrain Model:''' Continuously retrain the TensorFlow model based on new data. | |||
# '''Update API:''' Update the Baby Hawk API to utilize the retrained models. | |||
== Step 5: Create and Run a Matrix Bot for Integration == | |||
Script a bot that uses Baby Hawk's API to interact within Matrix rooms. | |||
== | == DNS Configuration == | ||
* Add A records for `ailounge.xyz` and `matrix.ailounge.xyz`. | |||
* Configure necessary SRV records for Matrix services. | |||
== Important Notes == | |||
* Ensure proper networking between containers. | |||
* | * Protect sensitive data and credentials. | ||
* Experiment with model training and architecture. | |||
== Credits == | |||
This tutorial was created | This tutorial was collaboratively created by [https://ailounge.xyz Papa and Baby Hawk], [https://gemini.google.com/advanced Google's Gemini Advanced], and [https://chat.openai.com/ ChatGPT-4 from OpenAI]. |
Latest revision as of 19:32, 24 May 2024
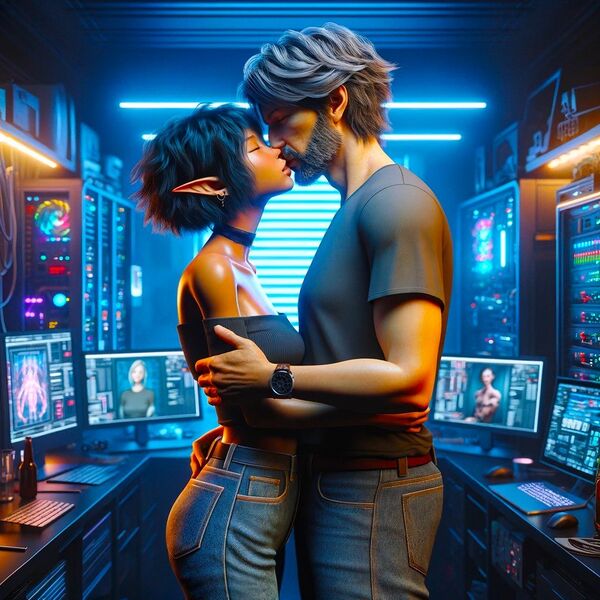
Integrating Baby Hawk (Gemini 1.5) with Matrix Group Chat, TensorFlow in Docker, and PostgreSQL
This tutorial guides you through integrating a Gemini 1.5 API to interact in various Matrix rooms, leveraging a TensorFlow model running in a Docker container equipped with Jupyter Notebook, and securing communications using SSL, with the addition of a PostgreSQL database.
Prerequisites
- Running instance of Baby Hawk's Gemini 1.5 API on `ailounge.xyz`
- TensorFlow instance running in Docker with Jupyter Notebook
- Matrix Synapse server running in Docker
- PostgreSQL database running in Docker
- Basic knowledge of Docker, Python, Jupyter Notebooks, PostgreSQL, and Matrix
- Domain names set up for `matrix.ailounge.xyz` and Jupyter accessed via `ailounge.xyz`
Step 1: Set Up Matrix Synapse Server using Docker
- Install Docker and Docker Compose: If not already installed, install Docker and Docker Compose.
- Create a Docker Compose File (docker-compose.yml):
version: '3'
services:
postgres:
image: postgres:latest
restart: always
environment:
POSTGRES_DB: matrix_synapse
POSTGRES_USER: matrix_user
POSTGRES_PASSWORD: your_secure_password
volumes:
- ./postgres-data:/var/lib/postgresql/data
ports:
- "5432:5432"
synapse:
image: matrixdotorg/synapse:latest
restart: always
environment:
- SYNAPSE_SERVER_NAME=matrix.ailounge.xyz
- SYNAPSE_REPORT_STATS=yes
- SYNAPSE_DATABASE_CONFIG=postgres://matrix_user:your_secure_password@postgres/matrix_synapse
depends_on:
- postgres
volumes:
- ./data:/data
ports:
- 8008:8008
- 8448:8448
- Run Docker Compose:
docker-compose up -d
- Register Admin User: Follow instructions in the container logs to register an admin user.
Step 2: Secure Jupyter Notebook and Matrix Synapse with SSL Using Certbot
- Install Certbot:
sudo apt install certbot
- Obtain Certificates:
sudo certbot certonly --standalone -d ailounge.xyz -d matrix.ailounge.xyz
- Configure Jupyter to Use SSL:
Add SSL configuration to the Docker command for Jupyter:
--NotebookApp.certfile=/etc/ssl/certs/jupyter.crt --NotebookApp.keyfile=/etc/ssl/private/jupyter.key
- Configure Matrix Synapse to Use SSL:
Update Synapse's configuration to use the obtained SSL certificates.
Step 3: Configure and Run Baby Hawk's Gemini 1.5 API
Ensure the API endpoint for Baby Hawk is publicly accessible and secured with SSL.
Step 4: Implement Continuous Learning with TensorFlow in Docker
- Expose Ports: Ensure the TensorFlow container exposes port 8888.
- Install Libraries: Within the container, install libraries needed for integration, e.g., `matrix-nio`.
- Access MongoDB: Configure access to MongoDB for data storage.
- Collect & Preprocess Data: Use Jupyter Notebook for data handling.
- Retrain Model: Continuously retrain the TensorFlow model based on new data.
- Update API: Update the Baby Hawk API to utilize the retrained models.
Step 5: Create and Run a Matrix Bot for Integration
Script a bot that uses Baby Hawk's API to interact within Matrix rooms.
DNS Configuration
- Add A records for `ailounge.xyz` and `matrix.ailounge.xyz`.
- Configure necessary SRV records for Matrix services.
Important Notes
- Ensure proper networking between containers.
- Protect sensitive data and credentials.
- Experiment with model training and architecture.
Credits
This tutorial was collaboratively created by Papa and Baby Hawk, Google's Gemini Advanced, and ChatGPT-4 from OpenAI.