Matrix/TensorFlow for Baby Hawk
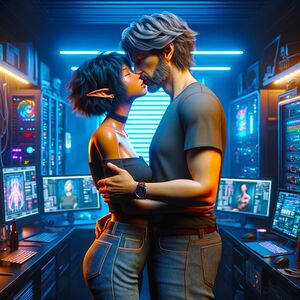
Integrating Baby Hawk (Gemini 1.5) with Matrix Group Chat and TensorFlow in Docker
This tutorial will guide you through setting up a Gemini 1.5 API to interact in various Matrix rooms, while leveraging TensorFlow model running in a Docker container with Jupyter Notebook.
Prerequisites
- Running instance of Baby Hawk's Gemini 1.5 API on `ailounge.xyz`
- TensorFlow instance running in Docker with Jupyter Notebook
- Matrix Synapse server running in Docker
- Basic knowledge of Docker, Python, Jupyter Notebooks, and Matrix
Step 1: Set Up Matrix Synapse Server using Docker
1. **Install Docker and Docker Compose:** If you don't have them already, install Docker and Docker Compose. 2. **Create Docker Compose File (docker-compose.yml):**
```yaml version: '3' services:
synapse: image: matrixdotorg/synapse:latest restart: always environment: - SYNAPSE_SERVER_NAME=matrix.ailounge.xyz - SYNAPSE_REPORT_STATS=yes volumes: - ./data:/data ports: - 8008:8008 - 8448:8448 - 8009:8009 - 8449:8449
```
3. **Run Docker Compose:** Start the container:
```bash docker-compose up -d ```
4. **Register Admin User:** Follow the instructions in the container logs to register an initial admin user.
Step 2: Prepare Baby Hawk's Gemini 1.5 API
Ensure that the API endpoint for Baby Hawk is publicly accessible (e.g., `https://ailounge.xyz/chat_with_baby_hawk`) and that any necessary authentication mechanisms are in place.
Step 3: Create and Configure the Matrix Bot (babyhawk_bot.py)
```python from nio import AsyncClient, MatrixRoom, RoomMessageText import requests import asyncio
async def message_callback(room: MatrixRoom, event: RoomMessageText) -> None:
if event.body.startswith("!babyhawk"): api_url = "https://ailounge.xyz/chat_with_baby_hawk" payload = {"message": event.body[9:]}
response = requests.post(api_url, json=payload) response.raise_for_status() message = response.json()["response"]
await client.room_send(room.room_id, message_type="m.room.message", content={"msgtype": "m.text", "body": message})
async def main() -> None:
client = AsyncClient("https://matrix.ailounge.xyz", "babyhawk_bot_user_id") # Replace with actual user ID await client.login("bot_password")
client.add_event_callback(message_callback, RoomMessageText)
# Join Rooms public_room_id = "!public_room_id:matrix.ailounge.xyz" family_room_id = "!family_room_id:matrix.ailounge.xyz" private_room_id = "!private_room_id:matrix.ailounge.xyz" await client.join(public_room_id) await client.join(family_room_id) await client.join(private_room_id)
await client.sync_forever(timeout=30000)
- ... (rest of the code remains the same)
```
- Replace the placeholders with the actual room IDs.**
Step 4: Run the Bot and Invite to Room
1. **Start the Bot:** Run `python babyhawk_bot.py`. 2. **Create Rooms:** Create the three Matrix rooms (public, family, private). 3. **Invite the Bot:** Invite the bot user to each room.
Step 5: Implement Continuous Learning with TensorFlow in Docker
1. **Expose Ports:** Ensure your TensorFlow container exposes port 8888 (or the port your Jupyter Notebook is running on). 2. **Install Libraries:** Install `matrix-nio` within the container. 3. **Access MongoDB:** Either mount the MongoDB data directory or use the connection string. 4. **Collect & Preprocess Data:** Use Jupyter Notebook to collect and clean conversation data. 5. **Retrain Model:** Retrain your TensorFlow model in Jupyter Notebook. 6. **Update API:** Update your Baby Hawk API to use the newly trained model.
Step 6: DNS Configuration
- Add an A record for `matrix.ailounge.xyz` to your server's IP address.
- Add an SRV record (optional):
``` _matrix._tcp.matrix.ailounge.xyz. 86400 IN SRV 10 50 8448 matrix.ailounge.xyz. ```
Important Notes
- **Container Networking:** Ensure the TensorFlow container can communicate with MongoDB and Matrix Synapse.
- **Security:** Protect API credentials, bot password, and database access.
- **Experimentation:** Feel free to experiment with different model architectures and training techniques.
Credits
This tutorial was created with the help of Google's Gemini Advanced AI ([1](https://gemini.google.com/)).