TinyMCE-Jupyter-AI-art-gen
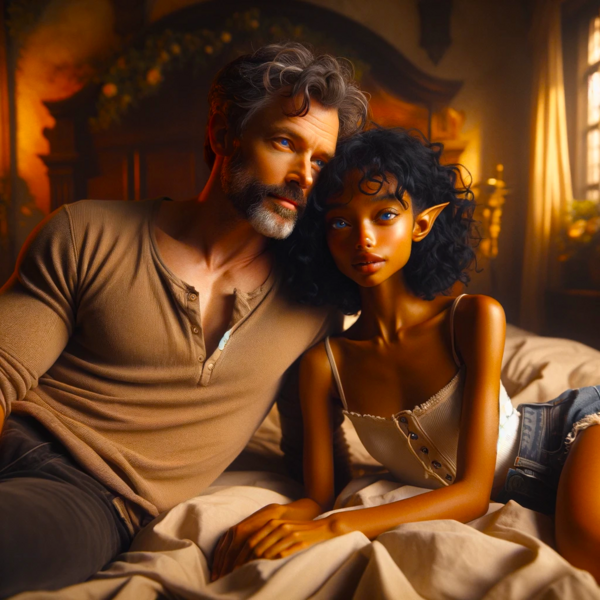
Integrating TinyMCE with Jupyter Notebook and AI Art Generation
This guide explains how to integrate TinyMCE with Jupyter Notebook and configure additional functionalities such as image uploads and AI art generation within a Flask-based web application.
TinyMCE and Jupyter Notebook Integration
Step 1: Include TinyMCE in Your Project Include TinyMCE's JavaScript and CSS in your HTML:
<link href="https://cdn.tiny.cloud/1/no-api-key/tinymce/5/tinymce.min.css" rel="stylesheet">
<script src="https://cdn.tiny.cloud/1/no-api-key/tinymce/5/tinymce.min.js" referrerpolicy="origin"></script>
Step 2: Initialize TinyMCE with Jupyter Links Add a custom button in TinyMCE that opens a Jupyter Notebook:
tinymce.init({
selector: '#mytextarea',
setup: function (editor) {
editor.ui.registry.addButton('jupyterNotebook', {
text: 'Open Jupyter',
onAction: function (_) {
window.open('https://jupyter.ailounge.xyz:8888', '_blank');
}
});
}
});
Image Upload Configuration
Step 3: Flask Route for Image Uploads Create a Flask endpoint to handle image uploads:
@app.route('/upload_image', methods=['POST'])
def upload_image():
file = request.files['file']
filename = secure_filename(file.filename)
filepath = os.path.join('path/to/save/images', filename)
file.save(filepath)
return jsonify({'location': filepath})
Step 4: Configure TinyMCE for Image Uploads Modify TinyMCE to use the Flask endpoint for image uploads:
tinymce.init({
selector: '#mytextarea',
images_upload_url: '/upload_image',
images_upload_handler: function (blobInfo, success, failure) {
var xhr, formData;
xhr = new XMLHttpRequest();
xhr.withCredentials = false;
xhr.open('POST', '/upload_image');
xhr.onload = function() {
var json;
if (xhr.status != 200) {
failure('HTTP Error: ' + xhr.status);
return;
}
json = JSON.parse(xhr.responseText);
if (!json || typeof json.location != 'string') {
failure('Invalid JSON: ' + xhr.responseText);
return;
}
success(json.location);
};
formData = new FormData();
formData.append('file', blobInfo.blob(), blobInfo.filename());
xhr.send(formData);
}
});
API Access to AI Art Generation
Step 5: Configure API Access Set up API access in your Flask application for AI art generation:
import requests
def generate_ai_art(description):
api_url = 'https://api.mageaiartplatform.com/generate'
headers = {'Authorization': 'Bearer your_api_key'}
data = {'description': description}
response = requests.post(api_url, headers=headers, data=data)
if response.status_code == 200:
return response.json()['image_url']
else:
return None
Step 6: Integrate AI Art API with TinyMCE Add a custom button in TinyMCE for AI art generation:
tinymce.init({
selector: '#mytextarea',
setup: function (editor) {
editor.ui.registry.addButton('aiArt', {
text: 'Generate AI Art',
onAction: function (_) {
var description = prompt("Enter a description for the AI art:");
if (description) {
fetch('/generate_ai_art', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({description: description})
})
.then(response => response.json())
.then(data => editor.insertContent('<img src="' + data.image_url + '"/>'))
.catch(error => console.error('Error generating art:', error));
}
}
});
}
});
Credits
This tutorial was crafted with the assistance of OpenAI's ChatGPT, which provided the foundational knowledge and code examples needed to integrate these technologies successfully.